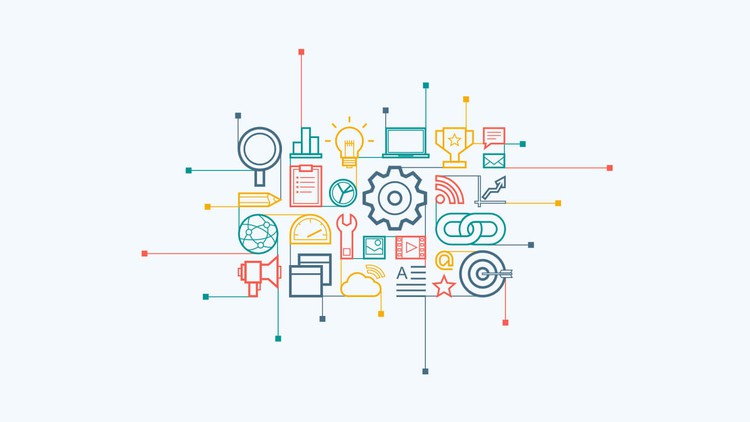
Description
Covers around 20+ most popular coding interview problems ranging from a variety of topics ( will have 200+ problems as 4 problems are being added every week)
- Merge Intervals
- Idea of Merge Intervals
- Merge Intervals
- Intervals Intersection (coming soon)
- Conflicting Appointments (coming soon)
- Two Pointers
- Idea of Two pointers and types of two pointers solutions
- Two Sum
- Remove Duplicates from Sorted Array
- 3 Sum
- Pair with Target Sum (coming soon)
- Squaring a Sorted Array (coming soon)
- Triplet Sum to Zero (coming soon)
- Triplet Sum Close to Target (coming soon)
- Triplets with Smaller Sum (coming soon)
- Subarrays with Product Less than a Target (coming soon)
- Dutch National Flag Problem (coming soon)
- Fast & Slow Pointers
- Linked List Cycle using Floyd’s Cycle Detection Algorithm
- Happy Numbers
- Ugly number (coming soon)
- Middle of the LinkedList (coming soon)
- LinkedList Cycle (coming soon)
- Start of LinkedList Cycle (coming soon)
- Bitwise Manipulation
- Single Numbers
- Flipping an Image
- Single Number II ( coming soon)
- Single Number III ( coming soon)
- Greedy Technique
- What are Greedy Algorithms ?
- Bulbs
- Highest Product
- Disjoint Sets
- Largest Permutation ( coming soon)
- Meeting rooms ( coming soon)
- Distribute Candy ( coming soon)
- Seats ( coming soon)
- Assign Mice to Holes ( coming soon)
- Majority Element ( coming soon)
- Gas Station ( coming soon)
- Island Patterns
- Intro to Matrix
- Number of Islands Explanation
- Number of Islands Code
- Number of distinct Islands Explanation
- Number of distinct island coding
- Count sub islands
- Max Area of an island (coming soon)
- Island Perimeter (coming soon)
- Flood fill (coming soon)
- Wall and Fates (coming soon)
- Minesweeper (coming soon)
- Surrounded Regions (coming soon)
- Find all groups of a farm land (coming soon)
- Topological Sort
- Introduction to Graph Problems
- Topological Sort
- Kahn’s Algorithm
- Implementing Topological Sort
- Course Schedule
- Alien Dictionary
- Minimum Height Trees (coming soon)
- All Ancestors of a Node in a Directed Acyclic Graph (coming soon)
- Build a Matrix With Conditions (coming soon)
- Find All Possible Recipes from Given Supplies (coming soon)
Upcoming Additions (3-4 problems are added every week)
- Sliding Window
- Easy
- Maximum Sum Subarray of Size K
- Smallest Subarray With a Greater Sum
- Medium
- Longest Substring with maximum K Distinct Characters
- Fruits into Baskets
- Hard
- Longest Substring with Distinct Characters
- Longest Substring with Same Letters after Replacement
- Longest Subarray with Ones after Replacement
- Easy
- Arrays
- Medium
- Group Anagrams
- Hard
- Trapping Rain Water
- Medium
- Merge Intervals
- Medium
- Intervals Intersection
- Conflicting Appointments
- Medium
- Linked List
- Easy
- Reverse a Linked List
- Medium
- Reverse a sub list
- Reverse a k-element sub list
- Easy
- Two Heaps
- Medium
- Find the median of a number stream
- Hard
- Maximum Capital
- Sliding Window Median
- Medium
- Top k elements
- Easy
- Top ‘K’ Numbers
- Kth Smallest Number
- ‘K’ Closest Points to the Origin
- Connect Ropes
- Medium
- Top ‘K’ Frequent Numbers
- Frequency Sort
- Kth Largest Number in a Stream
- ‘K’ Closest Numbers
- Maximum Distinct Elements
- Sum of Elements
- Hard
- Rearrange String
- Easy
- K-way merge
- Binary Search
- Backtracking
- 1D Dynamic programing
- 2D Dynamic programing
- Cyclic Sort
- Topological Sort
- Stacks
- Queues
- Depth First Search
- Breadth First Search
- Trie
- Hash Maps
- Math Tricks
- Miscellaneous
Who this course is for:
- Software Engineers who want to ace coding interviews in top tech firms
Requirements
- python
- basic idea of Data Structures and Algorithms
- Leetcode account
Last Updated 2/2023
Download Links
Direct Download
Data structure and algorithms for interviews.zip (863.6 MB) | Mirror
Torrent Download
Data structure and algorithms for interviews.torrent (49 KB) | Mirror